pythonでjsonファイルの読み書き
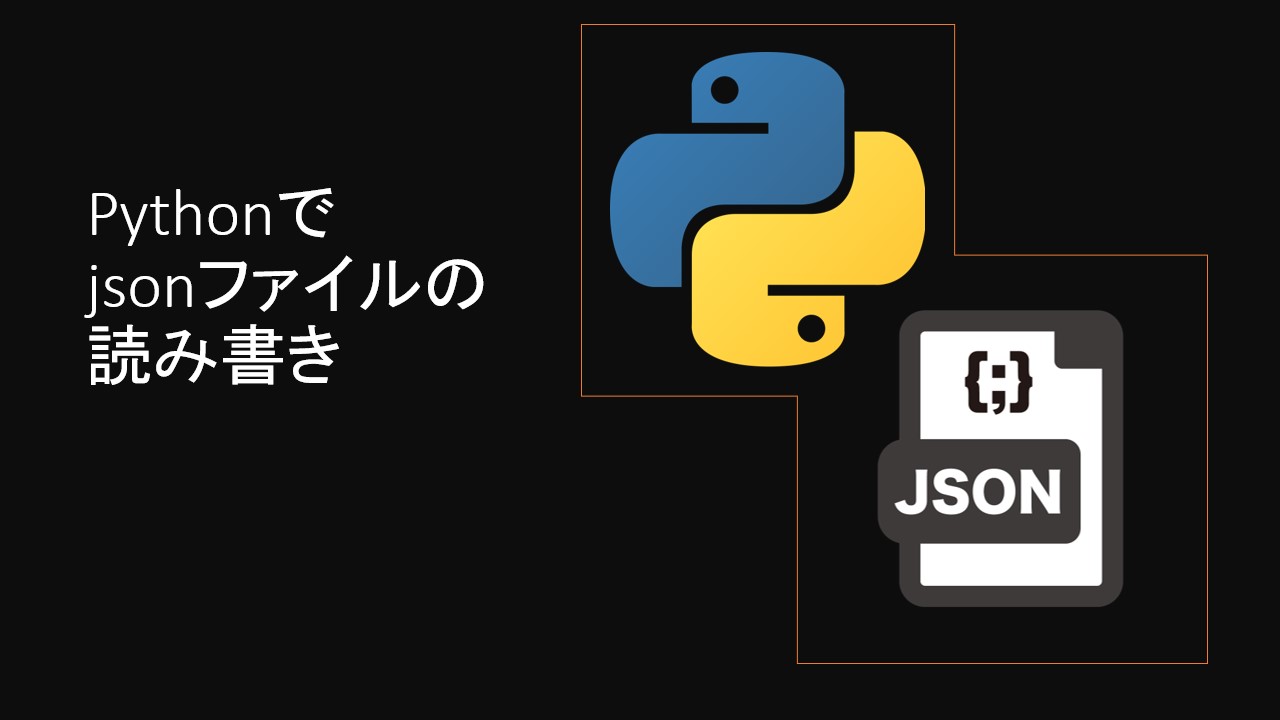
今回はpythonでjsonファイルの読み書きをしようと思います。
はじめに
ツールを作成する際、設定ファイルを用意しておいてツールに読み込ませて動作させることってありますよね。
pythonで設定ファイルを扱う場合、jsonファイルにしておけば辞書型でそのまま扱えるので便利だなと思い、使い方をメモ代わりに残しておきます。
JSONファイル操作
JSONファイルの基本
JSON (JavaScript Object Notation)は「JavaScriptのオブジェクトの書き方を元にしたデータ定義方法」のことです。
JSONの構造は、前述の通りpythonの辞書型と同じで{KEY:VALUE}の形式で各要素を定義します。
{
"str": "aaa",
"int": 10 ,
"list":[1,2,3,"bbb"]
}
ライブラリ
pythonの標準ライブラリであるjsonを使用します。
import json
JSON形式の文字列を辞書型に変換
json.loadを使用することで、JSON形式の文字列を辞書型に変換できます。
import json
f = open("test.json" , "r")
json_dict = json.load(f)
print(json_dict)
print(type(json_dict))
print(type(json_dict["str"]))
print(type(json_dict["int"]))
print(type(json_dict["list"]))
上記コードの出力結果は以下です。
{'str': 'aaa', 'int': 10, 'list': [1, 2, 3, 'bbb']}
<class 'dict'>
<class 'str'>
<class 'int'>
<class 'list'>
辞書型をJSON形式の文字列へ変換
json.dumpを使用することで、辞書型をJSON形式の文字列へ変換できます。
import json
f = open("test.json" , "r")
json_dict = json.load(f)
json_dict["int"] = json_dict["int"]*10
with open('test.json', mode='wt', encoding='utf-8') as file:
json.dump(json_dict, file, ensure_ascii=False, indent=4)
引数次第でインデントなどの細かいフォーマットを指定できます。詳細については以下参照。